We got some user feedback saying that it wasn’t totally clear to everyone that some of our content in the menus of our game was scrollable. We tried to make that more obvious by adding a bouncing animation for the content when the scrollable content is made visible.
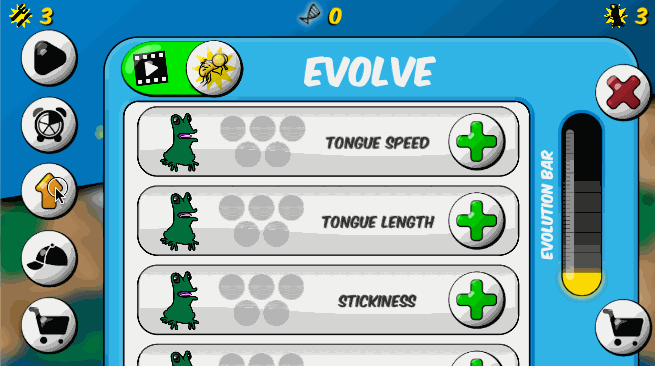
This is the code that does the magic. It’s hopefully pretty straightforward. You just add it in Unity as a script component and then you select which scrollbar you want to animate. You can change the bounce position and start/stop position to anything you would like (The value should be between 0 and 1). The SmoothTime will decide how long each of the animations will take.
Hopefully someone else out there will find this useful
using System; using UnityEngine; using UnityEngine.UI; public class BounceScrollAnimation : MonoBehaviour { public Scrollbar Scrollbar; public float BouncePosition = 0.95f; public float StartStopPosition = 1f; public float SmoothTime = 0.15f; float _currentVelocity; bool _animating; float _currentPosition; bool _bounced; void OnEnable() { _currentPosition = StartStopPosition; _animating = true; } void Update() { if(!_animating) return; if(!_bounced) Bounce (); else GoBack (); } void Bounce () { _currentPosition = Mathf.SmoothDamp (_currentPosition, BouncePosition, ref _currentVelocity, SmoothTime); Scrollbar.value = _currentPosition; if (Math.Abs (_currentPosition - BouncePosition) >= 0.001) return; _bounced = true; } void GoBack () { _currentPosition = Mathf.SmoothDamp (_currentPosition, StartStopPosition, ref _currentVelocity, SmoothTime); Scrollbar.value = _currentPosition; if (Math.Abs (_currentPosition - StartStopPosition) < 0.001) { _animating = false; _bounced = false; } } }